Enable SSL or HTTPS in IIS 7
1. Open IIS from Administrative Tools.
2. Navigate to Sites>Default Web Site. Or select the web site you want to enable SSL or HTTPS.
3. In the right pane, Click Binding.
4. Default is setup Port 80. You can click Add and to HTTPS or port 443.
5. Continue the instruction to complete the settigns.
Referencias:
[1]Adding HTTPS/SSL support to ASP.NET MVC routing
http://blog.stevensanderson.com/2008/08/05/adding-httpsssl-support-to-aspnet-mvc-routing/
[2]Requiring SSL For ASP.NET MVC Controllers
http://weblogs.asp.net/dwahlin/archive/2009/08/25/requiring-ssl-for-asp-net-mvc-controllers.aspx
[3]SSL pages under ASP.NET MVC
http://stackoverflow.com/questions/156748/ssl-pages-under-asp-net-mvc
[4]http://es.wikipedia.org/wiki/Hypertext_Transfer_Protocol_Secure
[5]Enabling SSL on IIS 7.0 Using Self-Signed Certificates http://weblogs.asp.net/scottgu/archive/2007/04/06/tip-trick-enabling-ssl-on-iis7-using-self-signed-certificates.aspx
[6] Configure IIS http://learn.iis.net/page.aspx/144/how-to-set-up-ssl-on-iis-7/
[7] IIS 7 SSL Certificate Installation http://www.digicert.com/ssl-certificate-installation-microsoft-iis-7.htm
[8] Enable SSL on IIS 7 (Spanish) http://thinkingindotnet.wordpress.com/2007/04/06/trucos-habilitar-ssl-en-iis-70-usando-certificados-firmados-por-nosotros/
[9] IIS7 - How to Install GoDaddy SSL Certificate http://www.netometer.com/video/tutorials/iis7-godaddy-ssl-certificate/
[10] Self-Signed Certificates on IIS 7 – the Easy Way and the Most Effective Way http://www.robbagby.com/iis/self-signed-certificates-on-iis-7-the-easy-way-and-the-most-effective-way/
Showing posts with label Windows.Developer. Show all posts
Showing posts with label Windows.Developer. Show all posts
Tuesday, March 22, 2011
Wednesday, December 15, 2010
ASP.NET C# Upload from URL
using System.Net;
WebClient wc = new WebClient();
wc.DownloadFile("http://sourcefile.ext", "drive:\\path\\targetfile.ext");
References:
[1] http://www.csharpfriends.com/Articles/getArticle.aspx?articleID=115
WebClient wc = new WebClient();
wc.DownloadFile("http://sourcefile.ext", "drive:\\path\\targetfile.ext");
References:
[1] http://www.csharpfriends.com/Articles/getArticle.aspx?articleID=115
Labels:
ASP.NET MVC,
C#,
Windows.Developer
Thursday, December 02, 2010
SQL Server Analysis Service
Working with Microsoft Analysis Services (SSAS) / MSOLAP / MDX Queries
http://developer.klipfolio.com/developer/cookbook_item/item-64
Running the Analysis Services Deployment Wizard
http://msdn.microsoft.com/en-us/library/ms174817.aspx
Building a SQL Server Analysis Services .ASDatabase file from a Visual Studio SSAS Project
http://agilebi.com/ddarden/2009/05/31/building-a-sql-server-analysis-services-asdatabase-file-from-a-as-project/
Resources
[1] http://sqlsrvanalysissrvcs.codeplex.com/
[2] SQL Server software
http://www.todotegusta.com/2010/05/microsoft-sql-server-2008-r2-standard-edition-x86-y-x64/
http://developer.klipfolio.com/developer/cookbook_item/item-64
Running the Analysis Services Deployment Wizard
http://msdn.microsoft.com/en-us/library/ms174817.aspx
Building a SQL Server Analysis Services .ASDatabase file from a Visual Studio SSAS Project
http://agilebi.com/ddarden/2009/05/31/building-a-sql-server-analysis-services-asdatabase-file-from-a-as-project/
Resources
[1] http://sqlsrvanalysissrvcs.codeplex.com/
[2] SQL Server software
http://www.todotegusta.com/2010/05/microsoft-sql-server-2008-r2-standard-edition-x86-y-x64/
Wednesday, December 01, 2010
Data Mining with C#
Data Mining with C# and ADO.NET
http://www.devsource.com/c/a/Languages/Data-Mining-with-C-and-ADONET/
Data Mining SDK
http://datamining.codeplex.com/
http://www.sqldev.org/sql-server-analysis-services/c-using-adomd-to-connect-to-olap-cube-10707.shtml
Another plataform
http://www.cs.waikato.ac.nz/ml/weka/
Other references:
Spanish
http://bernardorobelo.blogspot.com/2010/02/modelos-y-ejemplos-de-data-mining.html
http://alejandroesteban.wordpress.com/
http://www.devsource.com/c/a/Languages/Data-Mining-with-C-and-ADONET/
Data Mining SDK
http://datamining.codeplex.com/
http://www.sqldev.org/sql-server-analysis-services/c-using-adomd-to-connect-to-olap-cube-10707.shtml
Another plataform
http://www.cs.waikato.ac.nz/ml/weka/
Other references:
Spanish
http://bernardorobelo.blogspot.com/2010/02/modelos-y-ejemplos-de-data-mining.html
http://alejandroesteban.wordpress.com/
Wednesday, November 24, 2010
C# Smtp Client using gmail account
using System;
using System.Collections.Generic;
using System.Text;
using System.Net.Mail;
using System.Net;
namespace SmtpMail
{
class Program
{
static void Main(string[] args)
{
try
{
var client = new SmtpClient("smtp.gmail.com", 587)
{
Credentials = new NetworkCredential("compelligencelocal@gmail.com", "xxxxx"),
EnableSsl = true
};
client.Send("myusername@gmail.com", "manager_27@hotmail.com", "test", "testbody");
Console.WriteLine("Sent");
Console.ReadLine();
}
catch (Exception ex)
{
Console.WriteLine( ex.Message );
}
}
}
}
Friday, November 12, 2010
Postgres DEBUG
Errors and Messages
RAISE level 'format' [, expression [, ...]];
levels-> DEBUG, LOG, INFO, NOTICE, WARNING, and EXCEPTION(raises an error).
format-> string, % is replaced by the next optional argument's string representation.
usages:
RAISE NOTICE 'Calling cs_create_job(%)', v_job_id;
--This example replace % with v_job_id
RAISE EXCEPTION 'Nonexistent ID --> %', user_id;
--This example will abort the transaction with the given error message:
references:
[1] http://www.postgresql.org/docs/8.1/static/plpgsql-errors-and-messages.html
RAISE level 'format' [, expression [, ...]];
levels-> DEBUG, LOG, INFO, NOTICE, WARNING, and EXCEPTION(raises an error).
format-> string, % is replaced by the next optional argument's string representation.
usages:
RAISE NOTICE 'Calling cs_create_job(%)', v_job_id;
--This example replace % with v_job_id
RAISE EXCEPTION 'Nonexistent ID --> %', user_id;
--This example will abort the transaction with the given error message:
references:
[1] http://www.postgresql.org/docs/8.1/static/plpgsql-errors-and-messages.html
Labels:
J2EE,
Java,
Linux.Developer,
Postgres,
Windows.Developer
Wednesday, November 10, 2010
NHibernate works without mapping
ISQLQuery query = Session.CreateSQLQuery("SELECT p.*, c.* FROM Product p Left JOIN Product_CustomField c on p.ProductId=c.ProductId");
query.SetResultTransformer(NHibernate.Transform.Transformers.AliasToBean(typeof(Product)));
IList resultList = query.List();
Notes:
Add CusTomField1..5 into Product Class,not need declared in .hbm.xml
Combine with ScalarFields
public class SqlRepository {
...
public IList ListEmployees() {
using (ISession session = _sessionBuilder.GetSession()) {
return session
.CreateSQLQuery(@"
SELECT No_ AS EmployeeNumber, [E-mail Login] AS Username
FROM Employees")
.AddScalar("EmployeeNumber", NHibernateUtil.String)
.AddScalar("Username", NHibernateUtil.String)
.SetResultTransformer(Transformers.AliasToBean())
.List();
}
}
}
Add nHibernate mapping at run time
ISessionFactory sf = new Configuration()
.AddFile("Product.hbm.xml")
.AddFile("Category.hbm.xml")
References:
[1] http://docs.jboss.org/hibernate/core/3.3/reference/en/html/querysql.html
[2] Store procedure http://www.martinwilley.com/net/code/nhibernate/sql.html
[3] Reduced wiring code needed for native sql http://swik.net/Hibernate/Hibernate+GroupBlog/Hibernate+3.1:+Reduced+wiring+code+needed+for+native+sql
[4] AddJoin http://es.efreedom.com/Question/1-1132059/NHibernate-problema-de-AddEntity-y-AddJoin
[5] Native SQL http://knol.google.com/k/fabio-maulo/nhibernate-chapter-14-native-sql/1nr4enxv3dpeq/17#
[6]Fluent NHibernate http://wiki.fluentnhibernate.org/Auto_mapping
[7]Dynamically load .hbm.xml http://www.codeguru.com/forum/showthread.php?t=474322
query.SetResultTransformer(NHibernate.Transform.Transformers.AliasToBean(typeof(Product)));
IList
Notes:
Add CusTomField1..5 into Product Class,not need declared in .hbm.xml
Combine with ScalarFields
public class SqlRepository {
...
public IList
using (ISession session = _sessionBuilder.GetSession()) {
return session
.CreateSQLQuery(@"
SELECT No_ AS EmployeeNumber, [E-mail Login] AS Username
FROM Employees")
.AddScalar("EmployeeNumber", NHibernateUtil.String)
.AddScalar("Username", NHibernateUtil.String)
.SetResultTransformer(Transformers.AliasToBean
.List
}
}
}
Add nHibernate mapping at run time
ISessionFactory sf = new Configuration()
.AddFile("Product.hbm.xml")
.AddFile("Category.hbm.xml")
References:
[1] http://docs.jboss.org/hibernate/core/3.3/reference/en/html/querysql.html
[2] Store procedure http://www.martinwilley.com/net/code/nhibernate/sql.html
[3] Reduced wiring code needed for native sql http://swik.net/Hibernate/Hibernate+GroupBlog/Hibernate+3.1:+Reduced+wiring+code+needed+for+native+sql
[4] AddJoin http://es.efreedom.com/Question/1-1132059/NHibernate-problema-de-AddEntity-y-AddJoin
[5] Native SQL http://knol.google.com/k/fabio-maulo/nhibernate-chapter-14-native-sql/1nr4enxv3dpeq/17#
[6]Fluent NHibernate http://wiki.fluentnhibernate.org/Auto_mapping
[7]Dynamically load .hbm.xml http://www.codeguru.com/forum/showthread.php?t=474322
Sunday, November 07, 2010
How to get Machine Remote Client information
Access to the network host mac address,CPU access to suppliers,...
http://www.hackchina.com/en/cont/82131
Three ways to get your MAC address.
http://www.codeguru.com/Cpp/I-N/network/networkinformation/article.php/c5451
Enterprise Logging for Distributed J2EE Applications
http://javaboutique.internet.com/tutorials/logging/index4.html
How do I get MAC address of a host? (JAVA)
http://www.kodejava.org/examples/250.html
Extract network card address(JAVA)
http://www.rgagnon.com/javadetails/java-0369.html
References:
http://mindprod.com/project/macaddress.html
http://www.hackchina.com/en/cont/82131
Three ways to get your MAC address.
http://www.codeguru.com/Cpp/I-N/network/networkinformation/article.php/c5451
Enterprise Logging for Distributed J2EE Applications
http://javaboutique.internet.com/tutorials/logging/index4.html
How do I get MAC address of a host? (JAVA)
http://www.kodejava.org/examples/250.html
Extract network card address(JAVA)
http://www.rgagnon.com/javadetails/java-0369.html
References:
http://mindprod.com/project/macaddress.html
Wednesday, October 27, 2010
Postgres configuration
classical paths
# vi /etc/postgresql/8.2/main/postgresql.conf
# vi /var/lib/pgsql/data/postgresql.conf
# vi /opt/Postgres/8.4/data/postgresql.conf
Enable Network:
listen_addresses = 'localhost' to listen_addresses = '*'
You must also have appropriate entries in pg_hba.conf for hosts and
authentication type. You could use something like:
#TYPE DATABASE USER ADDRESS METHOD
local all all password
host all all 127.0.0.1/32 password
host all all 192.168.1.0/24 password
Next line allow all ips
host all all 0.0.0.0/0 md5
# vi /etc/postgresql/8.2/main/postgresql.conf
# vi /var/lib/pgsql/data/postgresql.conf
# vi /opt/Postgres/8.4/data/postgresql.conf
Enable Network:
listen_addresses = 'localhost' to listen_addresses = '*'
You must also have appropriate entries in pg_hba.conf for hosts and
authentication type. You could use something like:
#TYPE DATABASE USER ADDRESS METHOD
local all all password
host all all 127.0.0.1/32 password
host all all 192.168.1.0/24 password
Next line allow all ips
host all all 0.0.0.0/0 md5
Sunday, October 24, 2010
Subversion (svn)
Delete parts of subversion history
http://robmayhew.com/delete-parts-of-subversion-history/
Subversion Commands and Scripts
http://www.yolinux.com/TUTORIALS/Subversion.html
Delete Subversion revision
http://www.eiben.weite-welt.com/2008/01/delete_subversion_revision/
How do I change the default author for accessing a local SVN repository?
http://stackoverflow.com/questions/45624/how-do-i-change-the-default-author-for-accessing-a-local-svn-repository
http://robmayhew.com/delete-parts-of-subversion-history/
Subversion Commands and Scripts
http://www.yolinux.com/TUTORIALS/Subversion.html
Delete Subversion revision
http://www.eiben.weite-welt.com/2008/01/delete_subversion_revision/
How do I change the default author for accessing a local SVN repository?
http://stackoverflow.com/questions/45624/how-do-i-change-the-default-author-for-accessing-a-local-svn-repository
Thursday, October 21, 2010
Postgres save images
CREATE TABLE images (name text, img bytea);
File file = new File("image.gif");
FileInputStream fis = new FileInputStream(file);
PreparedStatement ps = conn.prepareStatement("INSERT INTO images VALUES (?, ?)");
ps.setString(1, file.getName());
ps.setBinaryStream(2, fis, file.length());
ps.executeUpdate();
ps.close();
fis.close();
setBinaryStream(), transfers a set number of bytes from a stream.
setBytes(), use this method if the contents of the image was already in a byte[].
Retrieving image
PreparedStatement ps = conn.prepareStatement("SELECT img FROM images WHERE name=?");
ps.setString(1, "image.gif");
ResultSet rs = ps.executeQuery();
if(rs.next()) {
byte[] imgBytes = rs.getBytes(1);
}
rs.close();
}
ps.close();
You could have used a InputStream object instead.
Alternatively you could be storing a very large file and want to use the LargeObject API to store the file:
CREATE TABLE imagesLO (imgname text, imgOID OID);
To insert an image, you would use:
conn.setAutoCommit(false); // All LargeObject API calls must be within a transaction
LargeObjectManager lobj = ((org.postgresql.PGConnection)conn).getLargeObjectAPI();
//create a new large object
int oid = lobj.create(LargeObjectManager.READ | LargeObjectManager.WRITE);
//open the large object for write
LargeObject obj = lobj.open(oid, LargeObjectManager.WRITE);
// Now open the file
File file = new File("image.gif");
FileInputStream fis = new FileInputStream(file);
// copy the data from the file to the large object
byte buf[] = new byte[2048];
int s, tl = 0;
while ((s = fis.read(buf, 0, 2048)) > 0)
{
obj.write(buf, 0, s);
tl += s;
}
obj.close();
//Now insert the row into imagesLO
PreparedStatement ps = conn.prepareStatement("INSERT INTO imagesLO VALUES (?, ?)");
ps.setString(1, file.getName());
ps.setInt(2, oid);
ps.executeUpdate();
ps.close();
fis.close();
Retrieving the image from the Large Object:
conn.setAutoCommit(false);
// Get the Large Object Manager to perform operations with
LargeObjectManager lobj = ((org.postgresql.PGConnection)conn).getLargeObjectAPI();
PreparedStatement ps = con.prepareStatement("SELECT imgOID FROM imagesLO WHERE imgname=?");
ps.setString(1, "image.gif");
ResultSet rs = ps.executeQuery();
if (rs.next())
{
int oid = rs.getInt(1);//open the large object for reading
LargeObject obj = lobj.open(oid, LargeObjectManager.READ);
//read the data
byte buf[] = new byte[obj.size()];
obj.read(buf, 0, obj.size());
//do something with the data read here
obj.close();
}
rs.close();
}
ps.close();
JSP Pagination mySQL Postgres
Postgres
select * from ubigeos order by nombre limit 10 offset 0
mySql
select * from ubigeos order by nombre limit 0 , 10
References:
[1] http://www.easywayserver.com/blog/jsp-pagination/
[2] http://www.todoexpertos.com/categorias/tecnologia-e-internet/programacion/java/respuestas/889187/paginacion-con-jsp
select * from ubigeos order by nombre limit 10 offset 0
mySql
select * from ubigeos order by nombre limit 0 , 10
References:
[1] http://www.easywayserver.com/blog/jsp-pagination/
[2] http://www.todoexpertos.com/categorias/tecnologia-e-internet/programacion/java/respuestas/889187/paginacion-con-jsp
Tuesday, October 12, 2010
Wednesday, October 06, 2010
Sunday, September 12, 2010
VB6 C#.NET Interop
[1] Using .NET Controls in VB6 http://www.codeproject.com/KB/vb-interop/UsingDotNETControlsInVB6.aspx
[2] Interop Forms Toolkit 2.0 Tutorial http://www.codeproject.com/KB/vb-interop/VB6InteropToolkit2.aspx
[3] InteropForms ToolKit - Visual Studio 2008 http://blogs.msdn.com/b/vbteam/archive/2008/03/05/interopforms-toolkit-visual-studio-2008-edition.aspx
[2] Interop Forms Toolkit 2.0 Tutorial http://www.codeproject.com/KB/vb-interop/VB6InteropToolkit2.aspx
[3] InteropForms ToolKit - Visual Studio 2008 http://blogs.msdn.com/b/vbteam/archive/2008/03/05/interopforms-toolkit-visual-studio-2008-edition.aspx
Thursday, September 09, 2010
ReportViewer with ASP.NET/ASP.NET MVC in Local Mode
Source code of original tutorial How to create local reports RDLC featuring barcode images in ASP.NET[0] on [1].
And adapted for ASP.NET MVC on [2]
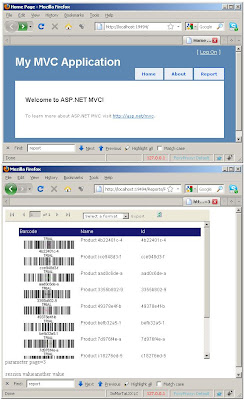
Reference
[0] http://neodynamic.com/ND/FaqsTipsTricks.aspx?tabid=66&prodid=1&sid=66#2
[1] ReportViewer ASP.NET Sample Local mode http://www.megaupload.com/?d=RMP2MMI1
[2] ReportViewer ASP.NET MVC Sample Local mode http://www.megaupload.com/?d=U4SCTYDG
[3]Understanding ASP.NET View State http://msdn.microsoft.com/en-us/library/ms972976.aspx
[4] Creating Charts Using Aspnet ReportViewer Control http://www.highoncoding.com/Articles/339_Creating_Charts_Using_Aspnet_ReportViewer_Control.aspx
[5] Microsoft Report Viewer Redistributable 2008 http://www.microsoft.com/downloads/en/details.aspx?familyid=cc96c246-61e5-4d9e-bb5f-416d75a1b9ef&displaylang=en
[6] Leveraging the ASP.NET ReportViewer Control http://www.devproconnections.com/article/aspnet2/leveraging-the-asp-net-reportviewer-control.aspx
And adapted for ASP.NET MVC on [2]
Reference
[0] http://neodynamic.com/ND/FaqsTipsTricks.aspx?tabid=66&prodid=1&sid=66#2
[1] ReportViewer ASP.NET Sample Local mode http://www.megaupload.com/?d=RMP2MMI1
[2] ReportViewer ASP.NET MVC Sample Local mode http://www.megaupload.com/?d=U4SCTYDG
[3]Understanding ASP.NET View State http://msdn.microsoft.com/en-us/library/ms972976.aspx
[4] Creating Charts Using Aspnet ReportViewer Control http://www.highoncoding.com/Articles/339_Creating_Charts_Using_Aspnet_ReportViewer_Control.aspx
[5] Microsoft Report Viewer Redistributable 2008 http://www.microsoft.com/downloads/en/details.aspx?familyid=cc96c246-61e5-4d9e-bb5f-416d75a1b9ef&displaylang=en
[6] Leveraging the ASP.NET ReportViewer Control http://www.devproconnections.com/article/aspnet2/leveraging-the-asp-net-reportviewer-control.aspx
Friday, May 14, 2010
Restaurando VS2008
Si perdiste tus templates los puedes restaurar asi
>devenv /ResetSettings
En mi caso fue asi.
>C:\Archivos de programa\Microsoft Visual Studio 9.0\Common7\IDE>devenv /resetset
tings
>devenv /ResetSettings
En mi caso fue asi.
>C:\Archivos de programa\Microsoft Visual Studio 9.0\Common7\IDE>devenv /resetset
tings
NHibernate
NHibernate y localización
http://codelog.climens.net/2010/03/16/nhibernate-y-localizacion/
http://codelog.climens.net/2010/03/16/nhibernate-y-localizacion/
Tuesday, April 06, 2010
Subscribe to:
Posts (Atom)
-
Resources: [1] Hela https://ome.grc.nia.nih.gov/iicbu2008/hela/index.html
-
Sunedu Renacyt 1) https://ctivitae.concytec.gob.pe/appDirectorioCTI/ 2) http://regina.concytec.gob.pe 3) https://www.gob.pe/9648-ser-p...
-
en inglĆ©s se llama “A potentially dangerous Request.Form value was detected from the client”. varias pĆ”ginas indican dos cosas: 1. agrega...
Running apps
Runtastic (I uninstalled because force to update your device - Internet connection problems) Runkeeper (Wrong GPS tracking) Strava (Curr...